myopenhab onlinestatus grabber
17.08.21 (Allgemein)
Wer viel im Smarthome bastelt möchte manchmal wissen warum etwas nicht mehr geht.
Ist die config nun falsch oder doch der Dienst mal wieder offline wegen dem Internet?
Openhab bietet zwar die Möglichkeit den zustand von Services und Things anzuzapfen, aber leider bekommt man darüber nicht heraus ob der Cloud Service gerade offline oder online ist.
Zu diesen zweck habe ich mir ein kleines PHP Scrip geschrieben das den „Cloud Status“ auf der Homepage abfragt und als json zu Verfügung stellt.
Vorbereitung: Installiere PHP und die benötigten Extensions:
Um dieses noch in openhab selber zu nutzen verwende ich ein exec Thing und php auf dem openhab host.
Im folgend nun eine Anleitung wie dies zu Installieren ist.
Als erstes die Abhängigkeiten installieren sudo apt install php-cli php-curl php-xml wget
und danach Installation prüfen:
php -m | egrep "xml|curl" curl libxml xml xmlreader xmlwriter
Skript nach "/etc/openhab/bin"
herunterladen (am Ende des Artikels auch nochmal verlinkt):
mkdir /etc/openhab/bin && wget https://gist.githubusercontent.com/carschrotter/4d3d26867edbf254097cd72fbf21a67f/raw/382c17bb9126b3db3e535998daee43a340691ea1/myopenhab_onlinestate.php -O "/etc/openhab/bin/myopenhab_onlinestate.php"
nun kann ein erster Testlauf starten:
sudo -u openhab /usr/bin/php "/etc/openhab/bin/myopenhab_onlinestate.php" --username="MYUSERNAME" --password="MYPASSWORD" --cookie="/etc/openhab/misc/COOKIE.TXT"
Dabei wird versucht euch auf myopenhab.org anzumelden und das Cookie mit der Session ID unter /etc/openhab/misc/COOKIE.TXT gespeichert. Im Anschluss solltet ihr noch die Benutzerrechte sowie Schreib,- und Leserechte anpassen chown root:openhab "/etc/openhab/misc/COOKIE.TXT"
und chmod 660 "/etc/openhab/misc/COOKIE.TXT"
Konfigurationsdatei anlegen:
nano "/etc/openhab/misc/myopenhab_conf.php"
und folgenden Inhalt einfügen:
„MYUSERNAME“ und „MYPASSWORD“ sind natürlich wieder durch eure Logindaten zu ersetzen.
Auch hier solltet ihr die Rechte anpassen chown root:openhab "/etc/openhab/misc/myopenhab_conf.php"
und chmod 640 "/etc/openhab/misc/myopenhab_conf.php"
Nun können wir schon mit dem einbinden in OpenHab anfangen. Dafür benötigt ihr das Exec Binding
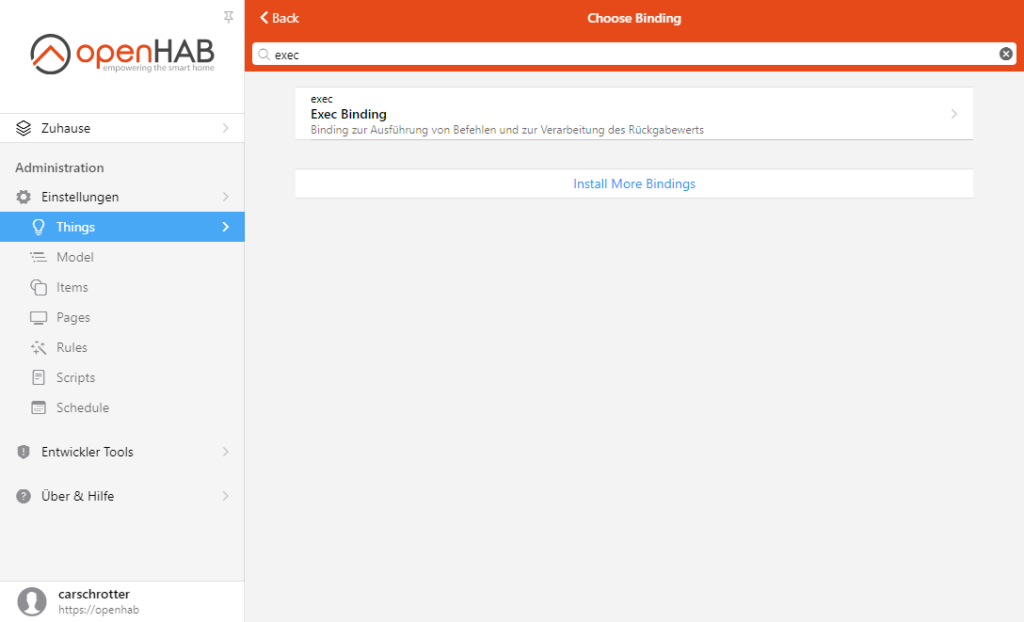
Nun erstellt ihr als erstes ein Exec Thing wenn ihr eine sprechende UID wollt müsst ihr diese beim erstellen angeben. Alle anderen Parameter können auch noch später z.B. auch im YAML geändert werden. Als Befehl habe ich /usr/bin/php "/etc/openhab/bin/myopenhab_onlinestate.php" --conf="/etc/openhab/misc/myopenhab_conf.php" --cookie="/etc/openhab/misc/COOKIE.TXT"
eingetragen. Den Status frage ich alle 3600 Sekunden ab also Jede Stunde ihr könnt aber auch 300 für z.B. alle 5 min eintragen.
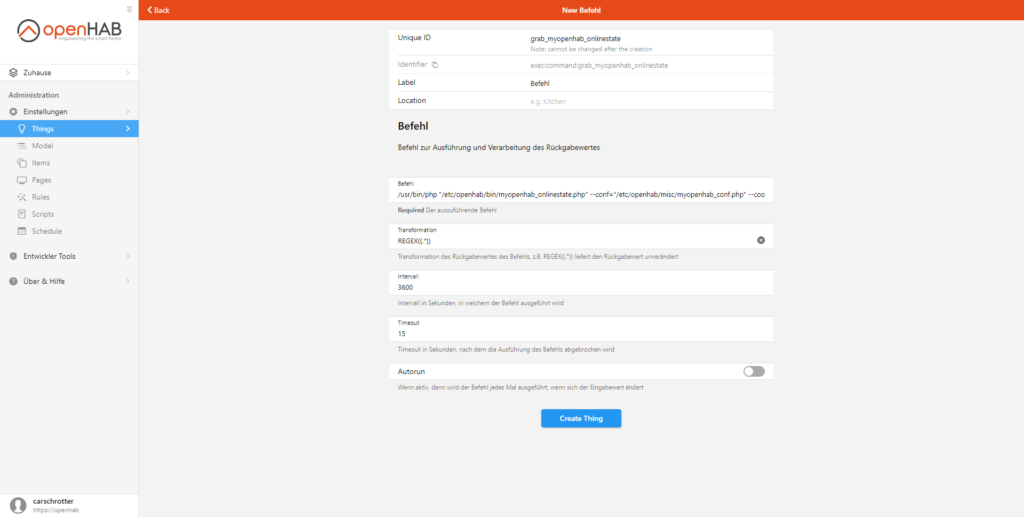
Nach den speichern könnt ihr auch das Thing als Code bearbeiten
UID: exec:command:grab_myopenhab_onlinestate label: myopenhab.org Status thingTypeUID: exec:command configuration: transform: REGEX((.*)) interval: 3600 autorun: true command: /usr/bin/php "/etc/openhab/bin/myopenhab_onlinestate.php" --conf="/etc/openhab/misc/myopenhab_conf.php" --cookie="/etc/openhab/misc/COOKIE.TXT" timeout: 15
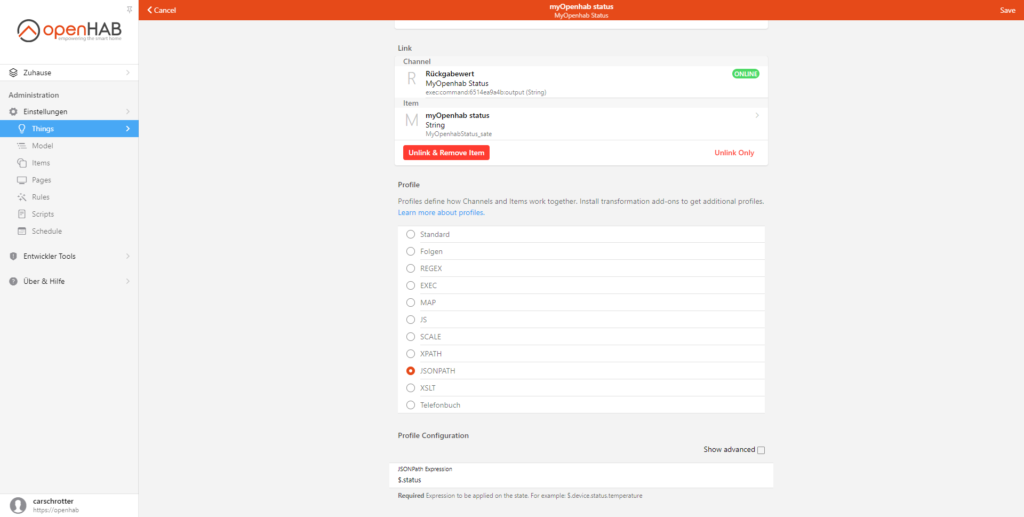